A Haar Cascade is an object detection algorithm.
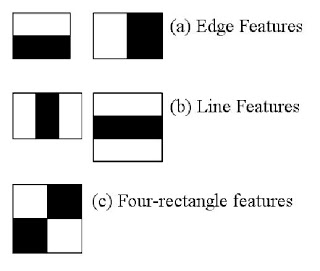
image courtesy of: https://docs.opencv.org
It uses a classifier which is trained to detect an object and does this by superimposing a positive image over a set of negative images over a number of stages. Quite a large number of stages.
This machine learning approach is computationally heavy and reliant upon possession of a training set. However there seem to be a number of them available online. Each with their own focus of object detection.
Haar-cascade Detection in OpenCV
OpenCV has it’s own trainer and detector which can be used to train your own classifier, which in our case would need to track the left eye of a person over a series of three images.
By applying some demo code kindly provided by OpenCV to one of our project images, we can see that this could well be a viable approach to take with our project and worthy of further research.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
import numpy as np import cv2 face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') eye_cascade = cv2.CascadeClassifier('haarcascade_eye.xml') img = cv2.imread('Ilovecats1.bmp') gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) faces = face_cascade.detectMultiScale(gray, 1.3, 5) for (x,y,w,h) in faces: cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2) roi_gray = gray[y:y+h, x:x+w] roi_color = img[y:y+h, x:x+w] eyes = eye_cascade.detectMultiScale(roi_gray) for (ex,ey,ew,eh) in eyes: cv2.rectangle(roi_color,(ex,ey),(ex+ew,ey+eh),(0,255,0),2) cv2.imshow('img',img) cv2.waitKey(0) # cv2.destroyAllWindows() |
Similar to HAAR Cascades are LBP (Local Binary Pattern) Cascades which seem to operate similarly in the respect that they both use training sets. However, LBP Cascades can provide quicker results with slightly less accuracy.
Local Binary Patterns could be thought of as a computationally cheaper version of the Haar Cascade. LBP works by thresholding with the use of a 3×3 grid. The value of the centre pixel in the grid is used as a threshold value and the surrounding pixels in the grid are thresholded and summed together to form the descriptor used. LBP yields results that would be similar to Haar Cascades while offering a much simpler process.
References:
Face Detection using Haar Cascades (accessed 25.10 2018)
https://docs.opencv.org/3.0-beta/doc/py_tutorials/py_objdetect/py_face_detection/py_face_detection.html#face-detection
Viola P. Jones MJ. Rapid Object Detection using a Boosted Cascade of Simple Features. IEEE Computer Society Conference on Computer Vision and Pattern Recognition 1:I-511- I-518 vol.1
https://www.researchgate.net/publication/3940582_Rapid_Object_Detection_using_a_Boosted_Cascade_of_Simple_Features
Deep Learning Haar Cascaded Explained (accessed 25.10 2018)
http://www.willberger.org/cascade-haar-explained/
Pietikäinen M. Local Binary Patterns. Machine Vision Group, Department of Electrical and Information Engineering, University of Oulu, Finland.
http://www.scholarpedia.org/article/Local_Binary_Patterns
Huang D. Shan C. Ardebilian M. Wang Y. Chen L. Local Binary Patterns and Its Application to Facial Image Analysis: A Survey
https://liris.cnrs.fr/Documents/Liris-5004.pdf